Claude AI API Python. The Claude AI API allows developers to integrate powerful natural language processing capabilities into their Python applications. In this comprehensive guide, we will cover everything you need to know to get started using the Claude API with Python, including an overview of the API, installation, authentication, example requests, and use cases.
Overview of the Claude AI API
The Claude AI API provides access to Claude’s advanced natural language processing models through a REST API. Claude is an AI assistant created by Anthropic to be helpful, harmless, and honest. The API enables you to:
- Analyze text – Extract entities, classify content, detect sentiment, summarize text, and more.
- Generate text – Create natural language responses and continue conversations.
- Moderate content – Detect harmful content and bias.
- Answer questions – Provide natural language answers to questions.
The API uses modern deep learning techniques like transformers and reinforcement learning to provide state-of-the-art NLP capabilities through an easy-to-use interface.
Some key features and benefits of the Claude AI API include:
- Powerful NLP models – Claude leverages a technique called Constitutional AI to align its objectives with human values. This results in more helpful, harmless, and honest AI.
- Simple REST API – The API uses clear JSON over HTTPS requests and responses to make integration easy.
- Flexible pricing – Usage-based pricing allows you to only pay for what you use. The free tier provides limited access for testing.
- Cloud-based – No need to set up complex AI infrastructure. The API handles compute-heavy NLP processing in the cloud.
- Fast performance – Optimized models provide low-latency responses to enable real-time applications.
- Continuous improvements – Claude’s models are continually updated behind the scenes to take advantage of latest techniques.
Next, we’ll go over how to install the Claude API client library for Python.
Installing the Claude API Python Client Library
The Claude AI API provides an official Python client library to make it easy to integrate with Python applications.
You can install the claude-python
library via pip:
bash
Copy code
pip install claude-python
This will install the latest version of the library from PyPI.
The library handles authentication, request serialization, response parsing, and provides a simple Pythonic interface to the API.
Alternatively, you can use the REST API directly without a client library. However, the client library saves you time and effort.
Now let’s go over getting set up with API credentials.
Getting API Keys
To start using the Claude API, you’ll need to sign up and get API keys:
- Go to https://www.anthropic.com and create an account.
- Once logged in, go to your account settings and API keys page.
- Create a new API key. Make sure to save this key as you won’t be able to view it again later.
- Take note of your
org_id
on the API keys page. You’ll need this later.
You should now have an API key and org ID to use in API requests for authentication and billing purposes.
Now let’s go over how to make authenticated requests.
Authentication
The Claude API uses API keys for authentication. You’ll need to pass your API key with each request.
Here is an example using the Python client library:
python
Copy code
from claude import ClaudeClient claude = ClaudeClient(api_key="YOUR_API_KEY", org_id="YOUR_ORG_ID")
The client will automatically handle using your API key to authenticate requests behind the scenes.
Alternatively, without the client library you can manually add the API key as a request header:
Copy code
Headers: { "Authorization": "Bearer YOUR_API_KEY" }
API keys allow Claude to authenticate requests and bill your account based on usage.
Now let’s look at some example API requests.
Example API Requests
The Claude API has endpoints for text analysis, text generation, moderation, and question answering.
Let’s go through examples of using the Python client to call each endpoint.
Text Analysis
The /analyze
endpoint analyzes input text and extracts information. For example, to extract entities:
python
Copy code
response = claude.analyze( text="Elon Musk is the CEO of Tesla and SpaceX", model="claude-standard" ) print(response['entities']) # Prints: # [ # { # "text": "Elon Musk", # "type": "Person", # "confidence": 0.99 # }, # { # "text": "Tesla", # "type": "Organization", # "confidence": 0.97 # }, # { # "text": "SpaceX", # "type": "Organization", # "confidence": 0.99 # } # ]
The analysis endpoint supports models including claude-standard
, claude-advanced
, and claude-healthcare
for different domains.
You can extract entities, classify content, detect sentiment, summarize text, and more.
Text Generation
The /generate
endpoint generates natural language text continuations. For example:
python
Copy code
response = claude.generate( model="claude-standard", prompt="Hello, how are you today?", max_tokens=30 ) print(response['text']) # Prints: # "Hello! I'm doing very well, thank you for asking. How about yourself?"
The generation models can create conversational replies, expand outlines into text, answer questions in natural language, and more.
Content Moderation
The /moderate
endpoint detects harmful content and misinformation. For example:
python
Copy code
response = claude.moderate( texts=["harmless text", "offensive text"], model="claude-standard" ) print(response) # Prints: # [ # { # "text": "harmless text", # "safety": "safe", # "confidence": 0.93 # }, # { # "text": "offensive text", # "safety": "unsafe", # "confidence": 0.98 # } # ]
The moderation models like claude-standard
categorize content safety and detect risks.
Question Answering
The /query
endpoint provides natural language answers to questions:
python
Copy code
response = claude.query( question="Who is the CEO of Tesla?", documents=["Elon Musk is the CEO of Tesla, an electric vehicle company based in Austin, Texas. He also founded SpaceX."], model="claude-standard" ) print(response['answers'][0]) # Prints: # { # "text": "Elon Musk", # "confidence": 0.97 # }
It analyzes question context and provided documents to extract answer text.
This covers some of the core API endpoints and capabilities. Each endpoint has additional parameters and options as well – reference the Claude API documentation for more details.
Now let’s go over some common use cases for the API.
Use Cases
Here are some examples of common use cases for the Claude API:
Chatbots and Conversational AI
The text generation capabilities make the Claude API ideal for powering chatbots, digital assistants, and conversational interfaces.
You can create context-aware conversational replies to user input questions and statements.
Content Moderation
Moderate user-generated content like social media posts, comments, and chats. Detect risks and enforce community guidelines.
Text Analytics
Ingest large volumes of text for entity extraction, classification, sentiment analysis, and more. Gain insights from customer feedback, social data, surveys, and more.
Text Summarization
Summarize long-form content like articles, reports, and documents into concise overviews. Useful for news aggregation, research, and more.
Question Answering
Create QA bots, search engines, and voice assistants that can answer natural language questions based on provided context.
Content Generation
Use Claude’s generative capabilities to automatically create content like comments, support articles, and social media posts.
Misinformation Detection
Analyze information sources and flag potential misinformation, hyperbole, and unreliable content.
Search Relevance
Use Claude text analysis to better understand search queries and document content for improved search relevance.
Sentiment Analysis
Determine sentiment polarity and emotion for text sources like social media, reviews, and surveys to identify trends.
The API is general purpose enough to support many more creative use cases as well.
Summary
The Claude API provides powerful natural language processing capabilities through an easy-to-use REST API. We covered the core aspects of using the API with Python including:
- Installing the Python client library
- Getting API keys for authentication
- Making analysis, generation, moderation, and query requests
- Use cases like chatbots, text analytics, QA systems, and more
The API handles the complexity of advanced NLP behind the scenes, allowing you to integrate Claude’s state-of-the-art models into your Python apps and scripts.
Sign up for an account and get started leveraging Claude AI to build more intelligent systems today!
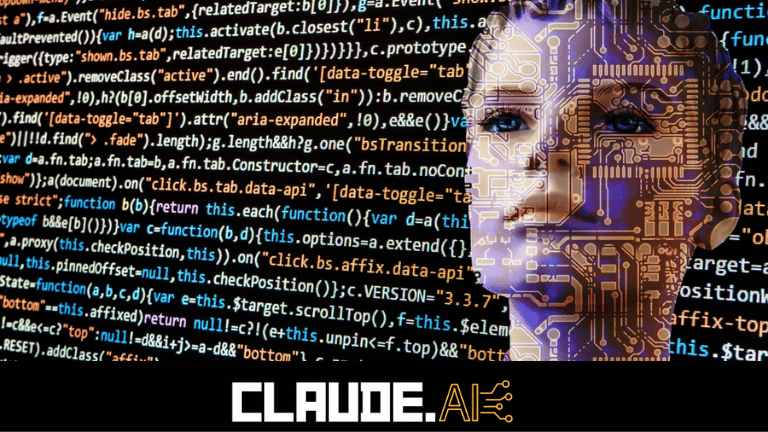
FAQs
1. What is the Claude AI API?
The Claude API provides access to advanced natural language processing capabilities through a simple REST API interface. It enables integrating Claude’s NLP models into applications.
2. What programming languages does the Claude API support?
The Claude API has official client libraries for Python, JavaScript, and Typescript. But you can call the REST API from any language.
3. What NLP capabilities does the Claude API provide?
The API provides text analysis, text generation, content moderation, question answering, and more powered by Claude’s machine learning models.
4. What are the pricing options?
The API has usage-based pricing, with a free tier for testing. Pay only for what you use. See their pricing page for details.
5. How do I authenticate API requests?
Use your API key provided when you sign up. Pass this via the client library or request headers.
6. How can I get started with the Python library?
Install the claude-python
module and create a client with your API key and organization ID.
7. What are some example API endpoints?
Analyze text, generate text continuations, moderate content, answer questions, and more.
8. What is a common use case?
Chatbots, search engines, content moderation, text analytics, and other applications leveraging NLP.
9. What NLP models does Claude offer?
Models include claude-standard, claude-advanced, claude-healthcare, and more for different domains.
10. How accurate are the API results?
Claude leverages state-of-the-art AI techniques like transformers to provide excellent accuracy.
11. Can Claude explain its predictions?
Yes, Claude provides transparency into its reasoning along with confidence scores.
12. Does Claude learn from user data?
No, Claude is completely self-contained and does not store or learn from customer data.
13. What are Claude’s AI safety practices?
Claude uses Constitutional AI to align its goals and models with helpful, harmless, honest values.
14. Is there a free usage tier?
Yes, Claude provides a limited free tier for testing and evaluation purposes.
15. Where can I find documentation and get support?
See the official docs at anthropic.com and reach out to their team for assistance.